How to generate HTML mailto links
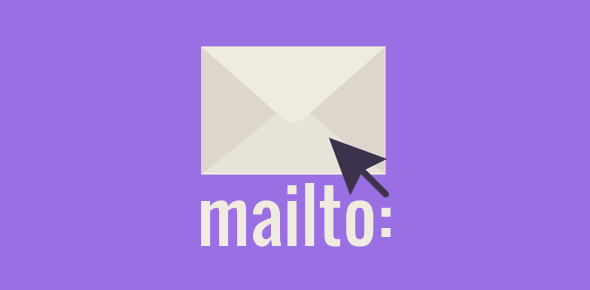
Generating HTML mailto links What is mailto link Mailto link is a type of HTML link that activates the default mail client on the computer for sending an e-mail. The web browser requires a default e-mail client software installed on his computer in order to activate the e-mail client. If you have Microsoft Outlook, for example as your default mail client, pressing a mailto link will open a new mail window. How to create mailto link in HTML Mail to email address with cc, bcc, subject and body <a href="mailto:rajatvedi007@gmail.com?cc=vedi.rajat@gmail.com&bcc=test@testing.com &subject=The%20subject%20of%20the%20email &body=The%20body%20of%20the%20email"> Send mail with cc, bcc, subject and body</a> Important Note - How to add spaces in the mail's subject or body You can add spaces by writing %20 in the text of the subject or body. <a href="mailto:test@gmail.com?subject=The%20subject&body=This%20is%20a%20message%20body">Send mail...